First Steps Building a Robot Car with Python & pyboard
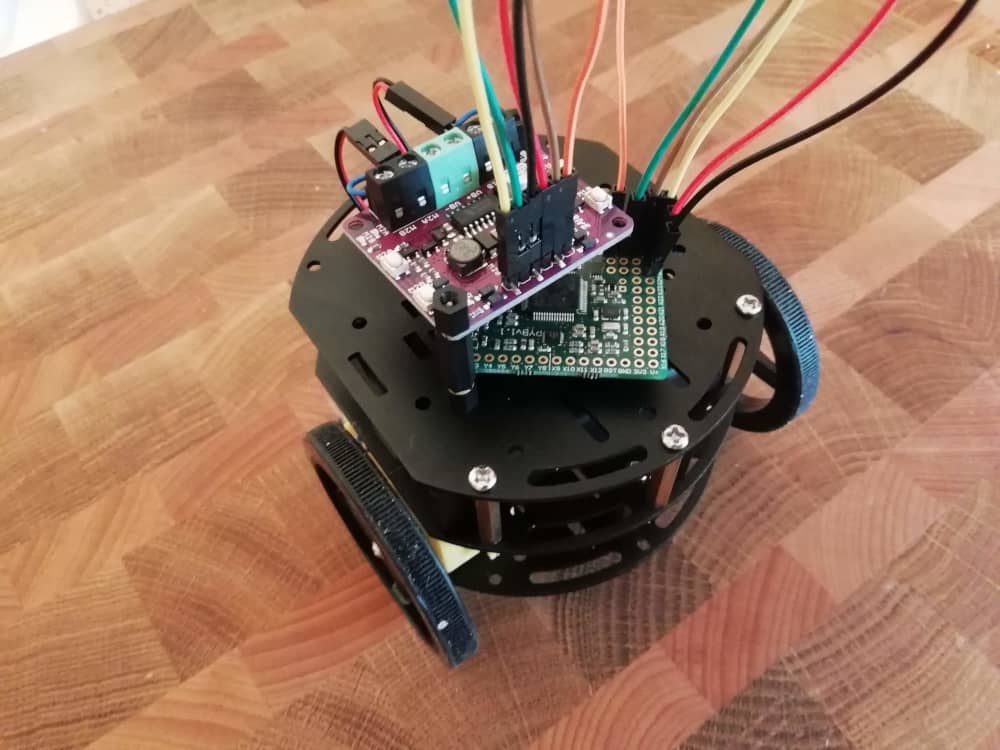
Required Components
- A chassis (I'm using this kit)
- pyboard
- Maker Drive motor driver
- Batteries (Example: 4 x AA batteries)
- 2 x DC Motors (If not included in your chassis kit)
Circuit Diagram
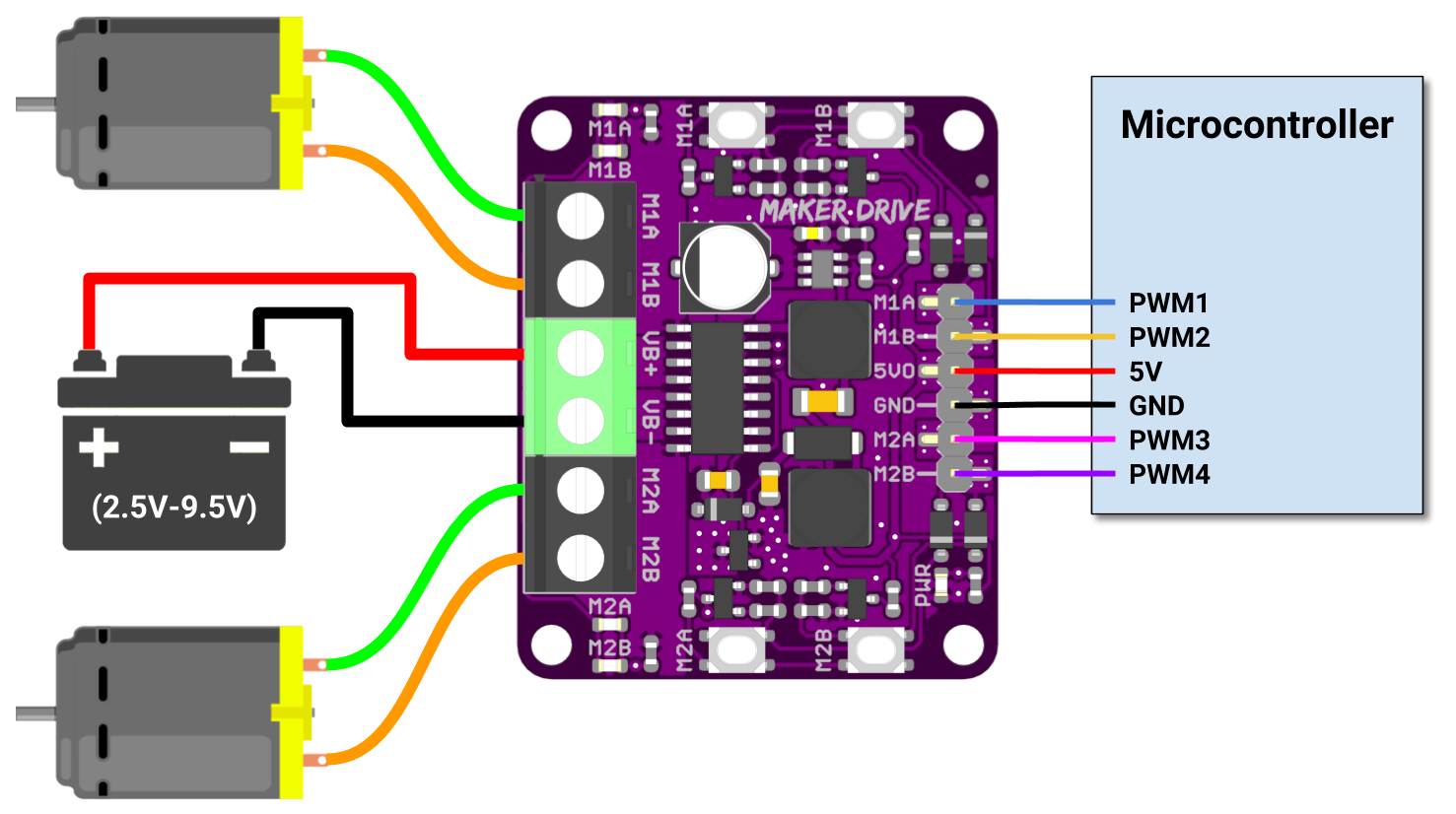
Code
The code is quite simple here. It will make the car run in a sort of a circle. You can see a video of it at the bottom of the article:
Click to see some code
from pyb import Pin, Timer import utime counter = 0 p1 = Pin('X1') # X1 has TIM2, CH1 p3 = Pin('X3') # X3 has TIM2, CH3 tim2 = Timer(2, freq=1000) ch1 = tim2.channel(1, Timer.PWM, pin=p1) ch3 = tim2.channel(3, Timer.PWM, pin=p3) while counter < 10: ch1.pulse_width_percent(50) ch3.pulse_width_percent(50) utime.sleep_ms(1000) ch1.pulse_width_percent(10) ch3.pulse_width_percent(50) utime.sleep_ms(500) counter += 1 ch1.pulse_width_percent(0) ch3.pulse_width_percent(0)
Updating pyboard firmware
I had owned the pyboard for a few years before I actually did something with it.
So, I suspected that the firmware might be out of date.
Therefore, the first item on the list is to update the firmware.
The documentation for this is excellent, but I did run into a minor problem because I kept downloading the firmware for pyboard v1.0, but I had v1.1. 🤦♂️ If you make sure you download the correct version of the firmware, you should be fine.
Running one motor
Once the pyboard is up to date, it's time to find out how to control one of the DC motors.
With the help of this article and the official documentation for PWM, the initial code to test running one motor looks like this:
from pyb import Pin, Timer
p1 = Pin('X1')
tim2 = Timer(2, freq=1000)
ch1 = tim2.channel(1, Timer.PWM, pin=p1)
ch1.pulse_width_percent(50)
Adding the second motor
With the first motor in place and running, adding the second motor is straightforward.
So after adding the second motor and making the relevant code changes, it looks like this:
from pyb import Pin, Timer
p1 = Pin('X1')
tim2 = Timer(2, freq=1000)
ch1 = tim2.channel(1, Timer.PWM, pin=p1)
ch1.pulse_width_percent(50)
p3 = Pin('X3')
ch3 = tim2.channel(1, Timer.PWM, pin=p3)
ch3.pulse_width_percent(50)
And voila!
Final touches
Once everything was working correctly, I put it together with the chassis and updated the code a bit to make it drive into a kind of a circle:
I'm really pleased with how well this went and honestly thought it would take longer.
Thank you for reading.